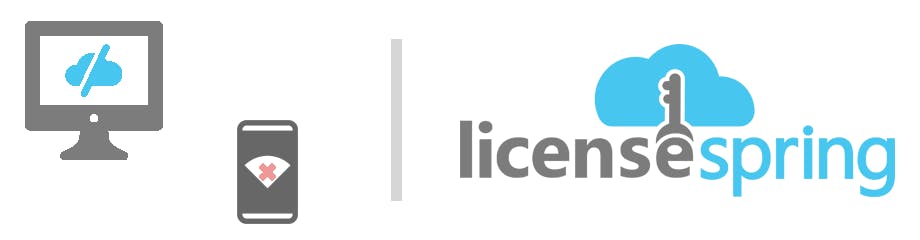
Discover how to streamline license management with out an active internet connection, covering activation, deactivation, total activations, license types, and common issues.
Unlike using a key-based license, user-based licenses depend on an email and password to activate a license. This tutorial will exhibit how to implement a login feature using the LicenseSpring SDK.
By the end of this tutorial, you should understand how to create a login feature by activating a license, how to create a logout feature by deactivating a license, and how to routinely perform online checks on your user-based license from your program. We will also consider the best practices for using a login mechanism within your application, creating a password reset feature, as well as LicenseSpring particularities between a trial and normal user-based licenses.
For illustration, we will create a ChatBot that operates as a simple login application. This tutorial will not cover Installing the SDK, Issuing Licenses, Offline activation, de-activation or checks.
Here is the repo for our login chatbot using the LicenseSpring C++ SDK.
Note: Since this article is a continuation of the initial tutorial, it is assumed that readers meet the prerequisites previously stated. Readers should also have a thorough understanding of license activation, deactivation, and checking.
Here are the steps we will go through:
Creating a login system with the user-based license is straightforward. You can ask the user to input their email and password combination. Once we have the user's credentials, we can then take that email/password combination and use it to activate our user-based license. How the user inputs their credentials is up to you.
1
2
3
4
std::string email = "UserInputtedEmail@example.com";
std::string password = "UserInputtedPassword";
auto licenseId = LicenseID::fromUser( email, password );
auto license = licenseManager->activateLicense( licenseId );
Once a user decides to log out, we can deactivate their license.
1
license->deactivate( true );
Note: deactivating a license on most SDKs will delete the local license file from your device. Some SDKs will have an optional parameter where you can decide whether to keep the local license file after deactivation. For information on where the local copy is stored on your device, see the Getting Started Tutorial.
It is recommended to perform a local license check at application open to confirm that the local license file belongs to the current device and has not been transferred. It is also useful to check whether the local license file has been tampered with and whether the local license is still valid. For more information on performing local license checks, see the Getting Started Tutorial.
1
2
license->localCheck(); //Perform a local (offline) check
license->check(); //Perform an online check
User data such as email address, first name, last name, license ID, order ID, and order store ID can all be retrieved through the SDK. Initial passwords are also available on your local license file, but once the password has been changed, you will no longer be able to access that data using the LicenseSpring SDK. All this user data is stored and encrypted on your local license file. The SDK allows you to retrieve the data and decrypt it. For more on license encryption/decryption see our tutorial on Safety/Security Considerations.
Here is how to retrieve a "User Data" object that contains such information
1
license->licenseUser();
Because activation spots on your license are limited, you may want to set a grace period on your application for users who are logged in, but are idle. To do this, the SDK provides offline tools that retrieve the date a license was last checked.
1
2
tm last_checked_date = license->lastCheckDate(); //Returns the date the license was last checked
int days = license->daysPassedSinceLastCheck(); //Returns the number of days since the last check
You can combine this with any method that routinely runs on an interval to see if a user has performed a license check within that interval. If they have not, you can log that user off by deactivating their license, freeing that activation spot for another user.
We can allow users to change their password. This can be done by the License Manager through the platform, but we can also allow end-users to change their password using our SDK. To change the password, we will require the original/old password and a new, replacement password. If the old password is correct, and the new password is valid, then the user's password will be changed. Note: this method requires internet connection.
1
license->changePassword( "old_passord", "new_passord" );
New accounts are created with a temporary, initial password. We can check if a license user is still using an initial password and prompt them to change their password using the following code:
1
2
3
4
5
auto user = license->licenseUser();
if ( user->isInitialPassword() )
{
//Prompt user to change password, use the change password method from the previous code block.
}
User-based trial licenses work differently from key-based trial licenses. Unlike key-based licenses, user-based trial licenses do not generate a new trial username/password combination like how key-based trial licenses generate new trial keys. User-based trial licenses require a valid email to be inputted before creating a trial license. If the account associated with that email is still using its initial password, then the trial license will automatically use that initial password to activate the trial license. If that account is not using its initial password, i.e. it has changed its password, then you will also need to set the password within the code using the SDK.
1
2
3
4
5
6
7
auto licenseId = licenseManager->getTrialLicense( "email@example.com" );
//The password field will be empty if the email account is not using an initial password.
if ( licenseId.password().empty() )
{
licenseId.setPassword( "password associated with email account" );
}
auto license = licenseManager->activateLicense( licenseId ); //returns a trial license
To learn more about Trial Licenses see the Trial License Tutorial.
You now have the basic tools and knowledge to set up your own login system using user-based licensing. It is important to note that this tutorial has only given you the bare minimum required to create a login system. It will be up to you to choose how you want to implement your login application, and how you will integrate LicenseSpring into said application. For a look at how a simple console login program can work using LicenseSpring and user-based licenses, take a look at our chatbot login sample code.